Reaching into your On-Prem services using Azure Hybrid Connections
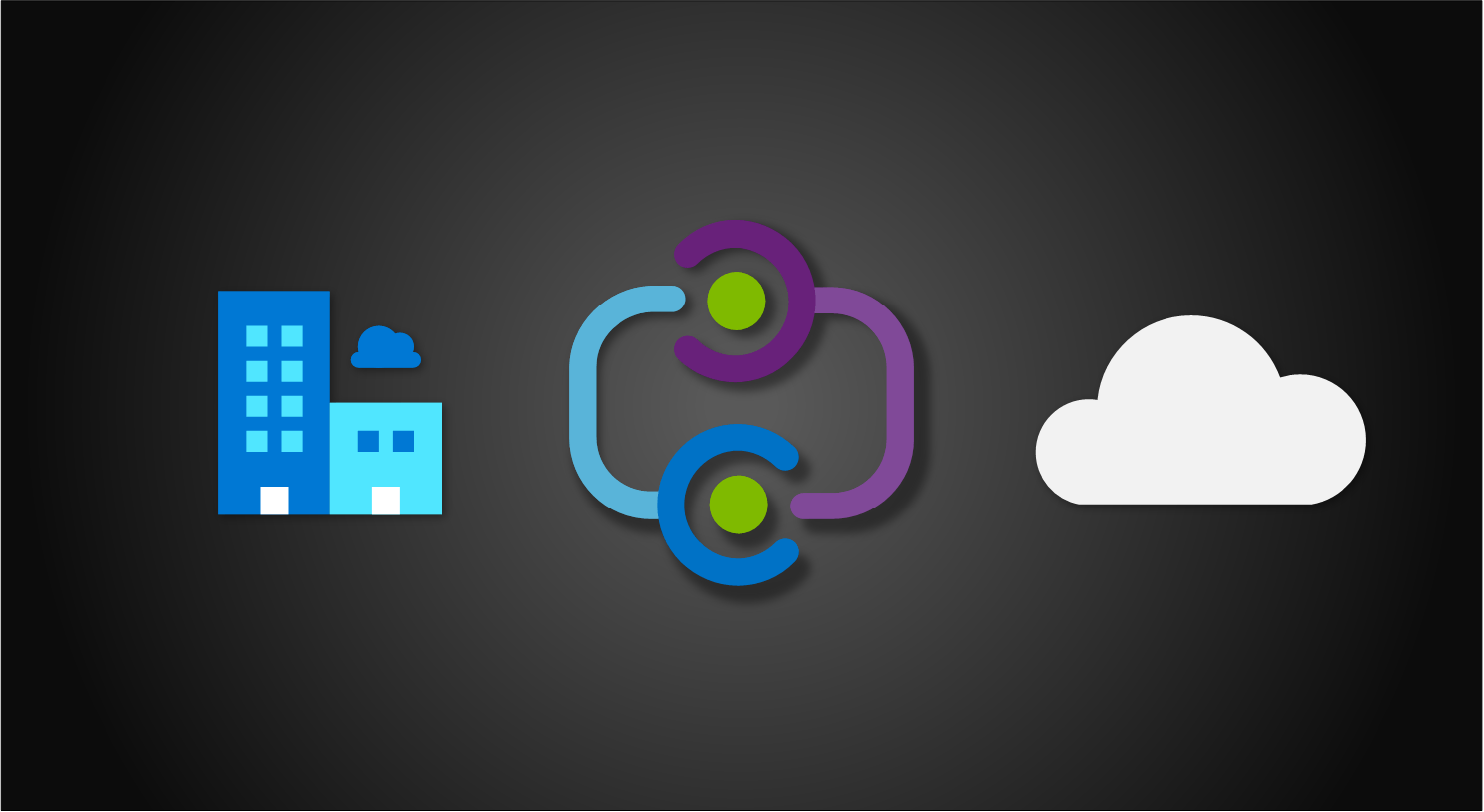
I recently used Azure Hybrid Connections on a client project and it turned out really well so I thought I’d share my experiences.
Previously I’ve used ngrok.io, Azure API Management and Azure Data Gateway but I found Azure Hybrid connections much easier to set up and use.
You can’t move every application to the cloud easily. But what if you want your cloud app to talk to your on-prem resources?
Azure Hybrid Connections enables you to connect your Azure App Services (including Azure Functions) to existing on-prem services/APIs.
Once configured with Hybrid Connections your Azure App Service (Web App) can make HTTP requests to on-prem API’s like https://servername/api/values or even http://localhost/api/values
How cool is that!!
Other uses for Azure Hybrid Connections?
Exposure
Normally you would have to talk to your System Admin to obtain a domain name or configure a sub-domain for your on-prem API that you want to expose to the Internet.
The Hybrid Connection Manager (HCM) is simpler to expose your API without either talking to your System Admin or obtaining a domain name.
Migration
Imagine a monolithic on-prem app with multiple microservices such as http://internal-northwind/api/customer or http://www.internal-northwind/orders.aspx that you want to expose to your Azure App Services.
I found using Hybrid Connections useful while migrating existing on-prem Web Apps and API’s to Azure one-by-one and still maintain connectivity with the private on-prem services. This is better than one monster migration.
Hybrid Connections aren’t just for exposing On-Prem services
Hybrid connections do not only apply to on-prem scenarios. They are just as useful for debugging App Services running in Azure.
Debugging - If one of your services is misbehaving you can host that service on your local machine running Visual Studio/Code. Once set up on your local machine you set up a Hybrid Connection on one of the calling App Services to route via the Hybrid Connection Manager (HCM) on your local machine. This will let you debug the service directly through Visual Studio and save the day.
What are the requirements for Azure Hybrid Connections
Azure Hybrid Connections can be used with any Azure App Service (including Azure Functions Apps) as long as the App Service Plan is not running on the Free Tier.
What is under the hood?
Microsoft Docs actually does a good job at explaining the inner workings of Hybrid Connections:
The Hybrid Connections feature consists of two outbound calls to Azure Service Bus Relay. There is a connection from a library on the host where your app is running in App Service. There is also a connection from the Hybrid Connection Manager (HCM) to Service Bus Relay. The HCM is a relay service that you deploy within the network hosting the resource you are trying to access.
Through the two joined connections, your app has a TCP tunnel to a fixed host:port combination on the other side of the HCM. The connection uses TLS 1.2 for security and shared access signature (SAS) keys for authentication and authorization.
When your app makes a DNS request that matches a configured Hybrid Connection endpoint, the outbound TCP traffic will be redirected through the Hybrid Connection.
How to use Azure Hybrid Connections
To demonstrate how easy it is to get Hybrid Connections set up and working we will follow these steps:
- On-Prem - Create an ASP.NET Core WebAPI and Deploy
- Cloud - Create a Functions App in Azure
- Cloud - Create and Configure the Hybrid Connection for the Azure Functions App
- Visual Studio - Code the the Azure Functions App and Deploy
- On-Prem Install the Hybrid Connection Manager (HCM)
- Cloud - Test the Azure Functions App is talking to the On-Prem API.
Done!
I give Azure Hybrid Connections 💎💎💎💎 out of 5 gems!
More information
1. On-Prem - Create an on-prem ASP.NET Core WebAPI
In this section we will create a simple ASP.NET Core WebAPI that will act as our on-premise service. This service will later be called from Azure via our Azure Functions App with a configured Hybrid Connection.
- Open Visual Studio 2019
- Create new project
- Select the ASP.NET Core Web Application template
- Choose API
- Untick Configure for HTTPS
WARNING - for this demo you must UNTICK Configure for HTTPS otherwise your connection will fail due to not having trust establised on both sides. In a future post I may show how to set up HTTPS and SSL for Azure App Services to connect to on-prem services that enforce TLS.
- Swap from IISExpess profile to the Kerstel profile
- F5 to start your API
- Navigate to http://localhost:5000/api/values
Great! Now we have a simple Web API that is running on-prem.
2. Cloud - Create a Functions App in Azure
Lets move onto creating an Azure Functions App that we will use to call our new on-prem API from Azure.
- Go to https://portal.azure.com
- Create Resource
- Select Compute
- Select Function App
- Fill in the details for the Functions App
- Make sure you select a Dedicated App Service Plan
- Click the Create button and wait for the app to be provisioned
3. Create and Configure the Hybrid Connection for the Azure Functions App
Finally, we can get to the fun part of creating and configuring the Hybrid Connection.
What we want is to create the Hybrid Connection and specify the endpoint of our on-prem API so that when the Azure Functions App makes an outbound call to the endpoint it will proxy through the Service Bus Relay and then the Hybrid Connection Manager to finally hit our endpoint.
- Go to the newly created Azure Functions App
- Click on Platform Features and the on Networking
3. Click on Configure your hybrid connection endpoints
- Click on Add hybrid connection
- Click on Create new hybrid connection
- Fill in the new connection endpoint details
- Click OK and notice that we now have a Hybrid Connection for our endpoint
4. Implementation the Azure Functions App
Here is some sample code for an Azure Functions App that makes a HTTP call to our simple on-prem API.
- Open Visual Studio
- Create a new project
- Select Azure Functions App project template
- Choose Empty functions template
- Right-click your project and select Add new item
- Select Class
- Call it HybridConnectionFunctions
- Paste the following code:
using System.Net.Http;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Azure.WebJobs;
using Microsoft.Azure.WebJobs.Extensions.Http;
using Microsoft.Extensions.Logging;
namespace HybdridConnectionAF
{
public static class HybridConnectionFunctions
{
private static readonly HttpClient HttpClient = new HttpClient();
[FunctionName(nameof(HybridCall))]
public static async Task HybridCall(
[HttpTrigger(AuthorizationLevel.Anonymous)]HttpRequestMessage req,
ILogger log)
{
HttpResponseMessage response = await HttpClient.GetAsync("http://localhost:5000/api/values");
string body = await response.Content.ReadAsStringAsync();
return new OkObjectResult(body);
}
}
}
- Press F5 to build and run the Azure Functions App locally (remember that our on-prem API should still be running)
- Test the Azure Functions App locally by sending a GET request to http://localhost:7071/api/HybridCall
- The response should match the JSON object returned from our on-prem API
WARNING: Right-click & Publish is not Best Practice! you are only allowed to do this if your company does not have a DevOps nit-picker!
- Right-click the Azure Functions project item
- Select Publish
- Click on Select Existing and click Publish
- Make sure you have the correct Azure account selected
- Browse through the available Resources dialog to find the Azure Functions App that we created earlier
- Select the Azure Functions App and click OK
Now we are ready to publish our Azure Functions App to the Azure Functions App Service we created earlier
- Click on the Publish button to publish our Azure Functions App
Wait for the Functions App to Build and Deploy to Azure
- Watch the Debug output window for any messages/warnings/errors
Now we are ready to Install the Hybrid Connection Manager to expose our local endpoint.
5. Install the Hybrid Connection Manager (HCM)
The Hybrid Connection Manager is the relay agent installed in/on the network that hosts our Hybrid Connection endpoint.
- From the Hybrid Connections page, click on Download connection manager
- Run the installer
- Open the Hybrid Connection Manager
- Click on Add a new hybrid connection
- When prompted, log into your Azure account
- Select your subscription from the dropdown (if you have more than one subscription)
- Click on the Hybrid Connection we created earlier and select Save
- Notice that our Hybrid Connection is listed in the main UI
6. Test the Published Azure Functions App
Test the Published Azure Functions App by:
- Sending a GET request to https://hybridconnectionapp.azurewebsites.net/api/HybridCall
- The response should match the JSON object returned from our on-prem API
If your response matches the expected output from your on-prem API then you have successfully set up Azure Hybrid Connections!
Awesome!
Troubleshooting Tips
All the tutorials and demo’s I found on the internet make it seem like the Hybrid Connections work immediately after adding them to the HCM. For me this was never the case. All my new connections always show up as Not Connected.
I simply have to restart the HCM (HybridConnectionManager) Service for my connections to work:
- Open Task Manager
- Select the Services tab
- Find HybridConnectionManager
- Right-click and Restart