Azure Functions v2 - Dependency Injection
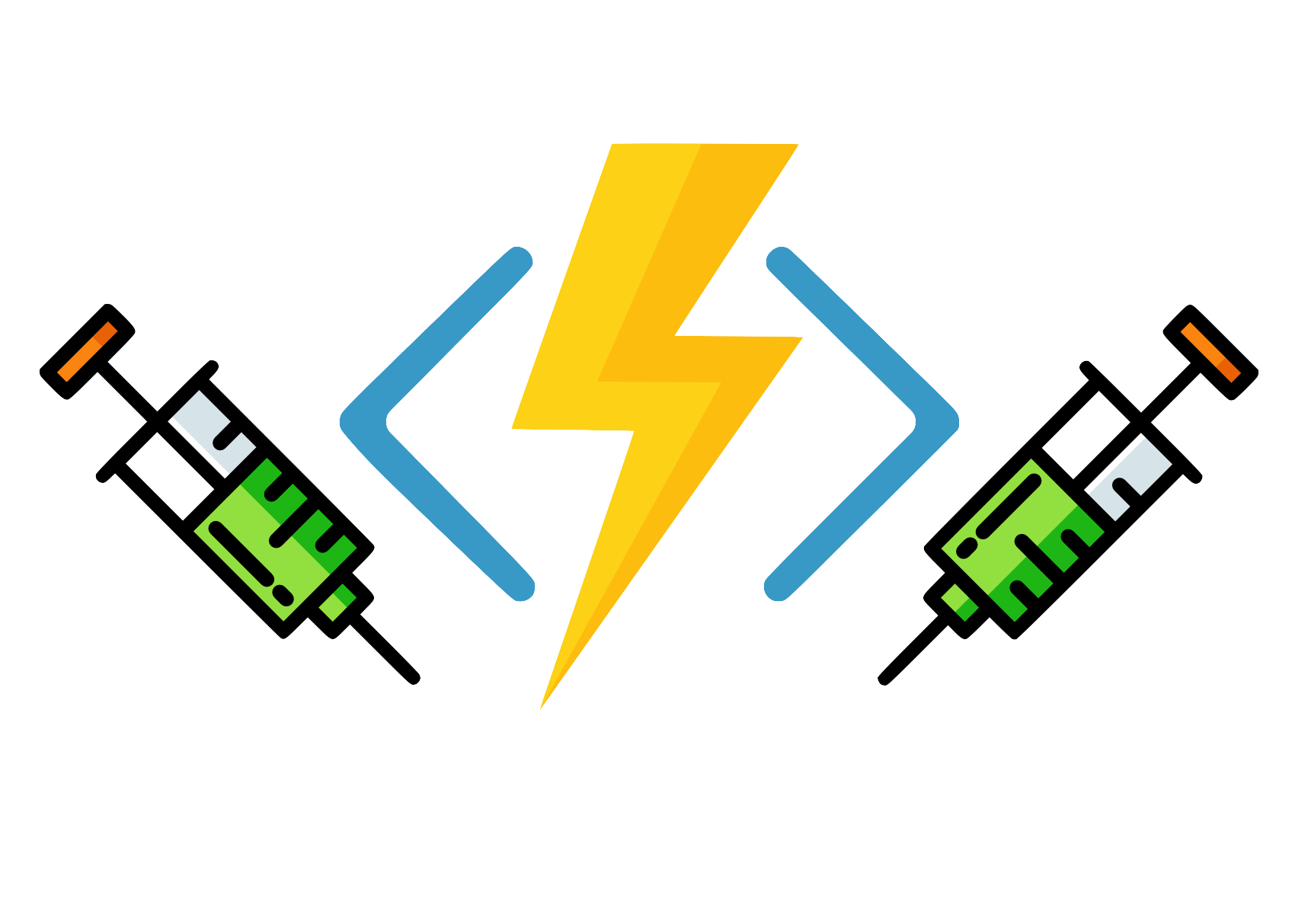
Dependency Injection for Azure Functions v2 was announced at Microsoft Build 2019. Check out my own Microsoft Build 2019 review.
Azure Functions v2 now provides built-in Dependency Injection. This new Dependency Injection feature will help us write cleaner, better abstracted and easier to test Azure Functions.
The Source Code for this post available on my GitHub repo.
All you need to get Dependency Injection working now is:
- add a reference the
Microsoft.Azure.Functions.Extensions
NuGet package - add a
StartUp
class that inherits from theFunctionsStartup
class - add an assembly setting to indicate which
FunctionsStartup
class to use - register some services using the
IFunctionsHostBuilder
Here is an example Startup
class:
using Microsoft.Azure.Functions.Extensions.DependencyInjection;
using Microsoft.Extensions.DependencyInjection;
// add this assembly annotation
[assembly: FunctionsStartup(typeof(DependencyInjectionDemo.Startup))]
namespace DependencyInjectionDemo
{
public class Startup : FunctionsStartup
{
public override void Configure(IFunctionsHostBuilder builder)
{
// register some services
builder.Services.AddSingleton();
}
}
}
Finally, using Constructor Injection you can inject your service into the Function class like this:
public class ConstructorInjectionDemo
{
private readonly ICustomService _svc;
public ConstructorInjectionDemo(ICustomService svc)
{
_svc = svc;
}
[FunctionName("ctorInjection")]
public IActionResult Run(
[HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route = null)] HttpRequest req,
ILogger log)
{
string message = _svc.GetMessage();
return new OkObjectResult(message);
}
}
You can set a breakpoint in the constructor and it will be hit when you call the api endpoint.
Currently, only Constructor Injection is supported. Trying to inject your services via Parameter Injection at the static entry point is not supported and causes binding errors.
Here is an example of Parameter Injection that will fail:
public class ParameterInjectionDemo
{
[FunctionName("paramInjection")]
public static IActionResult Run(
[HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route = null)] HttpRequest req,
ILogger log,
// inject our service as a parameter - this does not work!
ICustomService svc)
{
string message = svc.GetMessage();
return new OkObjectResult(message);
}
}
Check out the Documentation for some more examples.
I give Azure Functions Dependency Injection 💎💎💎💎 out of 5 gems!